How save time with the new Swift Charts feature in Swift WWDC 2022 brought new updates to the community-beloved native language for the Apple environment. Swift is back with a lot of improvements and new capabilities to help developers deliver world-class apps with ease by enhancing swift charts for data. In this blog, we’re checking […]
Introduction to Golang
Disclaimer
I am a layman who has just learned the Golang programming language for a few months at Icehouse. Therefore, take everything with a pinch of salt.
I wrote this material since I wanted to share and review my experiences about what I have learned in the Golang programming language. In addition to it, blogging about these materials can help me understand more about Golang.
Keep in mind as well that the material in these blogs might not be entirely up-to-date. Sometimes it is necessary to do further research for this material in order to obtain the latest and updated information. This material was also obtained based on the results of the author’s research. If you find an error in the material, I encourage you to directly let me know and I will be glad to correct the error.
What is Golang?
Golang, which is also known as Go, is a free and open-source programming language which was created and maintained by Google. It was created in 2007 by developers from Google: Robert Griesemer, Ken Thompson, and Rob Pike. Their purpose was to create a reliable program.
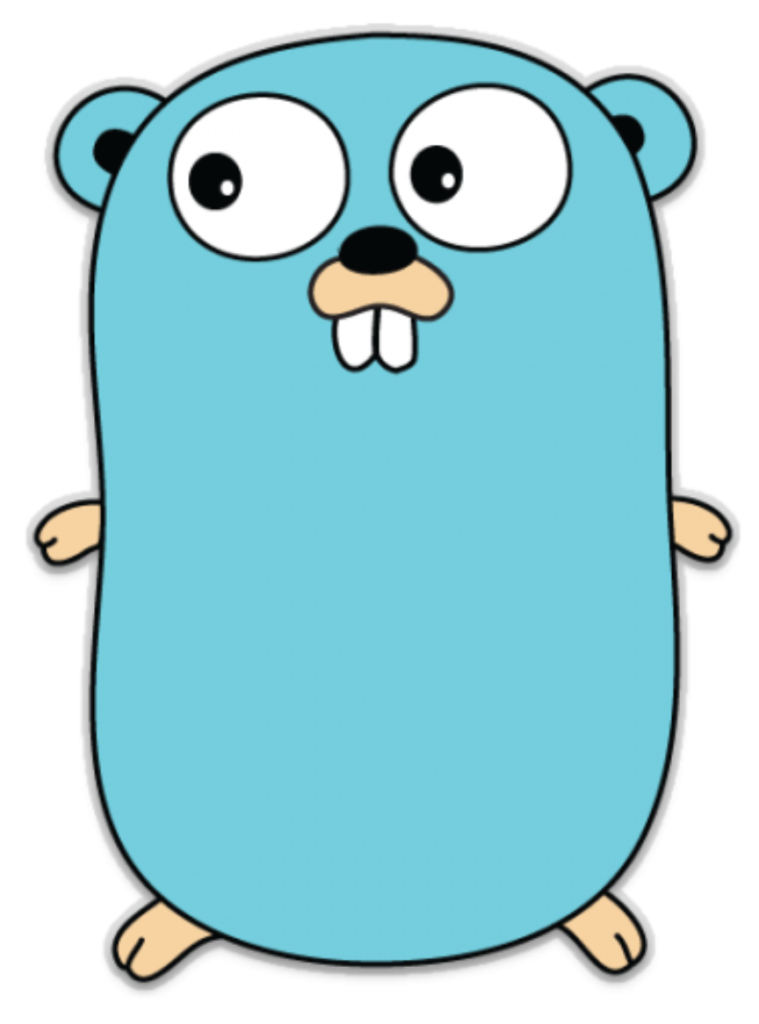
Go also has a mascot, Gopher, which is inspired by an animal called a platypus. Half of its blue body is shaped as an icon. From what you may know, Golang has recently increased in popularity; the programming language was at the peak of its journey in 2009 and 2016.
Features
- It is compiled, simple for beginners to start, concurrent, statically-typed, and efficient.
- Accommodates concurrency concepts.
- Strings are UTF-8 encoded by default.
- Has garbage collector and memory safety.
- Focused on handling system programming language, heavy-server centric web services, and text-processing problems.
Why Use Golang?
- Familiar and easy to learn: Go is a modified programming language from the C class. However, Go has a more concise syntax, therefore, learning Go is easier and faster. In addition, Go is also similar to Python and Ruby in nature.
- Meets developer needs: Go is here to meet the needs of developers in their daily lives. This language has an impact on software that runs fast and efficiently.
- Simplicity of server side: Go provides a built-in standard library which is the HTTP protocol to make it easier for developers to use.
- Statically typing system: Language that requires developers to explicitly define a data type when they create a piece of data.
- Similar to object-oriented language: Struct and object in Go, with the help of methods and pointers look alikes with the OO language.
Go Installation
The following are mandatory steps to install Golang on MacOS based on what the author has done:
- Go to the following link https://golang.org/dl/, choose the installation version and process the selected file type, for example ‘.pkg’.
- Open the package file and follow the instructions to install it.
- Verify the successful installation by using the ‘go-version’ command in the terminal, and make sure the version of Golang appears in the terminal.
Getting Start
Hello World and Running the App
Steps | Screenshot |
Starting from zero, the first step which developers always do each time they try a new language is to create a hello world program. | ![]() |
First, create a file named main.go and copy the code snippet above onto the file. Then run your program with ‘go run main.go’ in the terminal. You should see the output in the terminal as follows: | ![]() |
Getting User Input
The following shows an example of a simple code form with a main function that asks the user to input a string (scanln) and prints the input value (print).
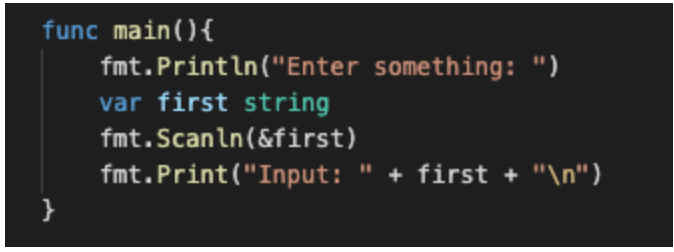
Working with Multiple Files
Sometimes coding can seem less efficient when all the code is written on one page. To show this, here is an example of a simple code created in separate files.
Steps | Screenshot |
A main file main.go, becomes the main part of the application when it is run. The body function has a derivative function called greet(). | ![]() |
On the other hand, developers also create a separate file called greet.go. The file has a greet() function that prints the words “Hello!” when executed properly. | ![]() |
Running the code in this situation is quite a tricky task. Many people may think that running this program can be done just by using the ‘go run main.go’ command, but in reality, it’s not that simple. To run more than one Go file, developers must include the name of the other files in the terminal as follows: ‘go run main.go greet.go’.
Creating a Simple HelloWorld HTTP Server
Again with the same idea but a little bit more advanced, This section will nudge towards the server API, discussing “How to Make a Simple HTTP” server that responds to the given string like “Hello World”.
Getting Package
Firstly, you should import the library functions the program needs. “fmt” is called for formatted input-output and “net/http” is called for network capabilities which deal with different functions provided for the web server category.
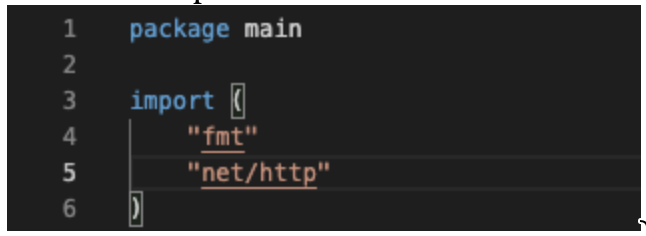
Adding HandleFunc Method
The next step is to add the HandleFunc method (with two parameters) inside the main function. The first parameter is a root directory and the second one is the function to be called.
The ListenAndServe method is called next to listen on the TCP network address and it will call the server which handles requests for an upcoming connection.
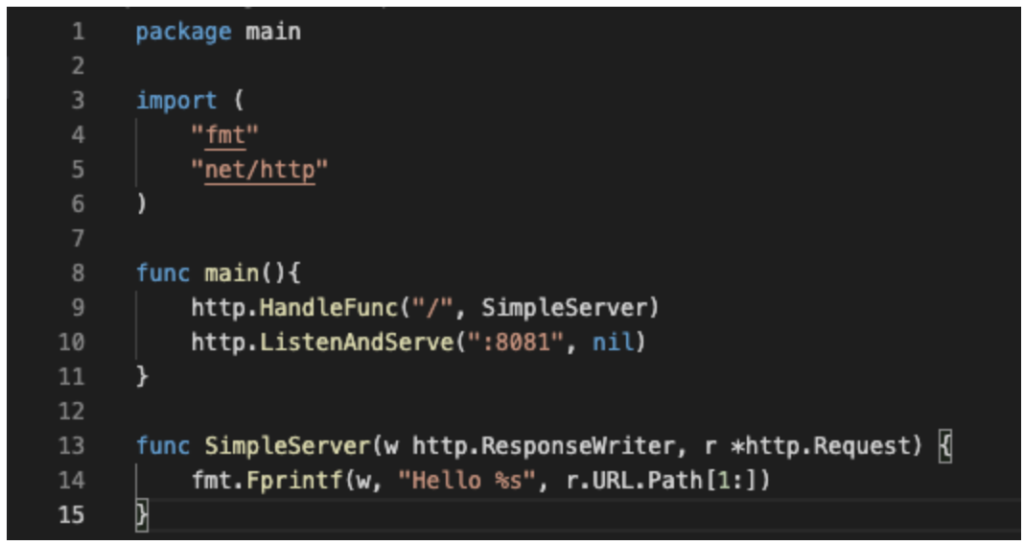
Adding SimpleServer Method
Last, but not least, is to create a SimpleServer method, which has two parameters, an HTTP response writer and an HTTP request. The response writer is provided by HTTP handler to construct HTTP response, and the request is of type pointer, symbolized by a star, to call the request (received by the server or sent by the client).
The code inside the function will print the formatted string which contains a response variable (w), a string and a request variable from the removed URL/path.
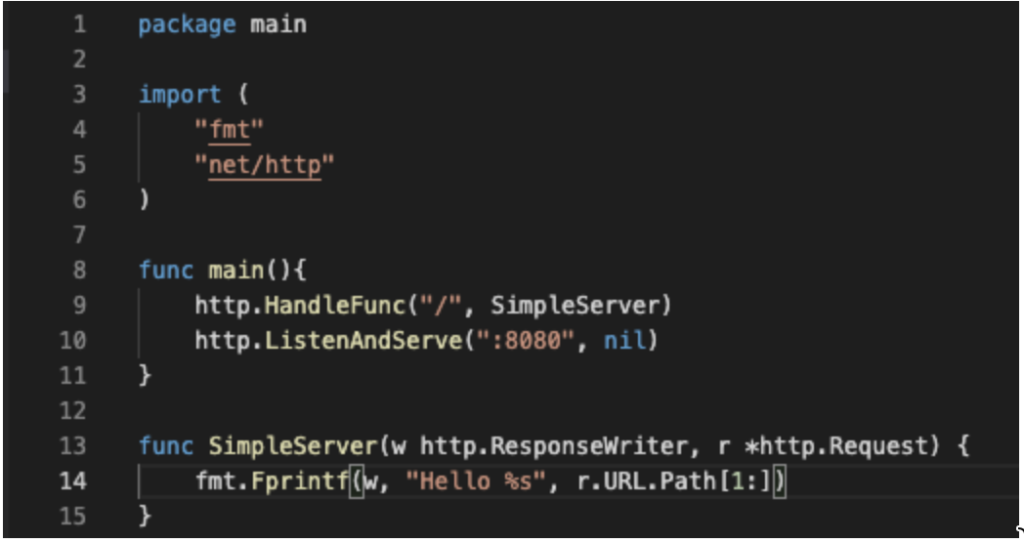
Running the SimpleServer
Run the command as in the image below to get the expected output from the system. | |
Since port 8081 was defined in the code, opening “localhost:8081” on the browser will result in the image below. | |
When adding a string to the root directory such as “Giannis”, the browser will automatically show the following output. |
Go Data Types
Golang has multiple data types divided into 4 parts:
- Basic type: Numbers, string, and booleans.
- Aggregate type: Array and struct.
- Reference type: Pointer, slices, maps, functions.
- Interface type: Data type is write by using the following format.

Go Control Structure
Operator
Operators are symbols which operate mathematical functions and logical manipulations. The following are the types of operators in Golang:
- Arithmetic Operator includes (+) as addition, (-) as subtraction, (*) as multiplication, (/) as division, (%) as modulo, (++) as increment, and (–) as decrement.
- Relational Operator includes (==) as equality, (!=) as not equal, (<) as lower than, (>) as greater than, (<==) as less than equal-to, and (>==) as greater than equal-to.
- Logical Operator includes (&&) as AND, (||) as OR, and (!) as NOT.
- Bitwise Operator includes (&) as AND, (|) as OR, (^) as XOR, (<<) as Left Shift, and (>>) as Right Shift
- Assignment Operator includes (=) as Simple, (+=) as Add AND, (-=) as Subtract AND, (*=) as Multiply AND, (/=) as Divide AND, (%=) as Modulo AND, (<<=) as Left Shift AND, (>>=) as Right Shift AND, (&=) as Bitwise AND, (^=) as bitwise exclusive OR, and (|=) as bitwise inclusive OR.
- Miscellaneous Operator includes (&) as address of variables, and (*) as a pointer.
Function
Functions are the basic building blocks of Go. A function declaration tells the compiler about the name, return type and parameters. Functions provide the actual body in a code. Functions aim to solve problems by dividing them into small and reusable tasks.
Function Name | Description | Screenshot |
Return a Value | The greet function returns a string type value. | |
Return Multiple Values | The animal function returns three values, a string, a string and an int. | |
If Else | If else statement serves to validate a condition which has previously been set, whether it returns to the desired result (if) or the other (else). | |
For Loop | this is an example of a simple loop in Go. The code prints the index number until the number of the index is 10. | |
For Loop As While Loop | Since there is no while loop keyword in Go, it can be achieved by removing the init and post statements in for loop, as shown in the following example. | |
Range Loop | A range loop can be defined by adding the range to replace the increment/decrement part. This kind of loop is usually used to iterate through maps, arrays, and strings. | |
Struct | Go allows the use of a struct, which is a user-defined type that allows a group of items with different types combined into a single type. This concept is generally compared with classes in OOP. It can also be determined as a lightweight class which only supports composition but not inheritance. |
Standard Library and Packages
Go has more than 250 built-in packages and the API is the same for all the systems. Each package has its own function and different uses. The following are examples of common libraries that are usually used in the simple coding process on Go.
- os/exec: gives the ability to run external OS commands and programs.
- syscall: low-level, external package, which provides a primitive interface to the underlying OS’s calls.
- fmt: contains functionality for formatted input-output.
- io: provides basic input-output functionality.
- bufio: wraps around io to give buffered input-output functionality.
- path/filepath: contains routine for manipulating filename paths targeted at the OS.
- strconv: converts strings to basic data types.
- unicode: special functions for unicode characters.
- regexp: for string pattern-searching functionalities.
Advanced Learning
This is a brief lesson on the basics of Golang. For further material, please refer to the official documentation at https://golang.org/doc/. Now, there are many available online courses about various topics of Go. In addition to it, the following are some examples of libraries/frameworks written in Go that might interest you:
- Gin, a HTTP web framework in Go (https://github.com/gin-gonic/gin),
- Sqlx, a library that provides a set of extensions for Go’s standard database/sql (https://github.com/jmoiron/sqlx),
- JWT Go, a JWT implementation in Go (https://github.com/golang-jwt/jwt),
- A Go implementation for uuid (https://github.com/twinj/uuid),
This is also great for developers to understand Go faster: (https://www.educative.io/blog/become-golang-developer).
Reference
https://www.educative.io/blog/golang-tutorial
https://medium.com/swlh/an-introduction-to-golang-7369339dba3
https://medium.com/rungo/introduction-to-go-programming-language-golang-89d16ca72bbf
https://betterprogramming.pub/go-4f365468dbd5
https://medium.com/gophersland/gopher-vs-object-oriented-golang-4fa62b88c701
More Articles
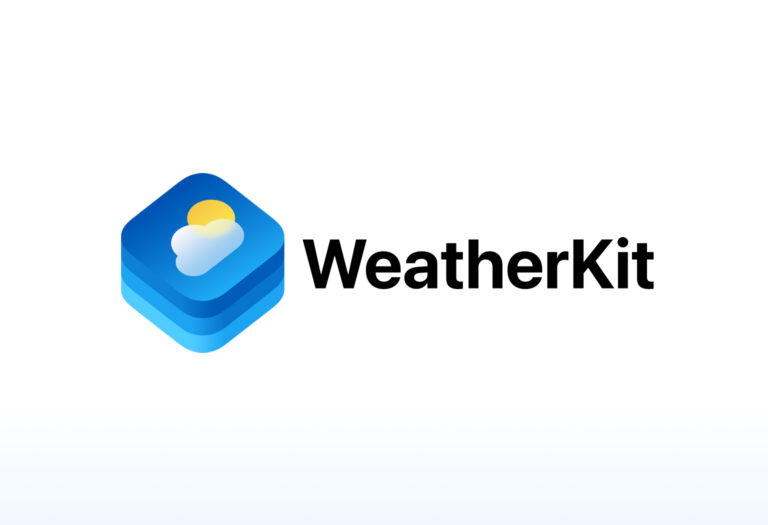
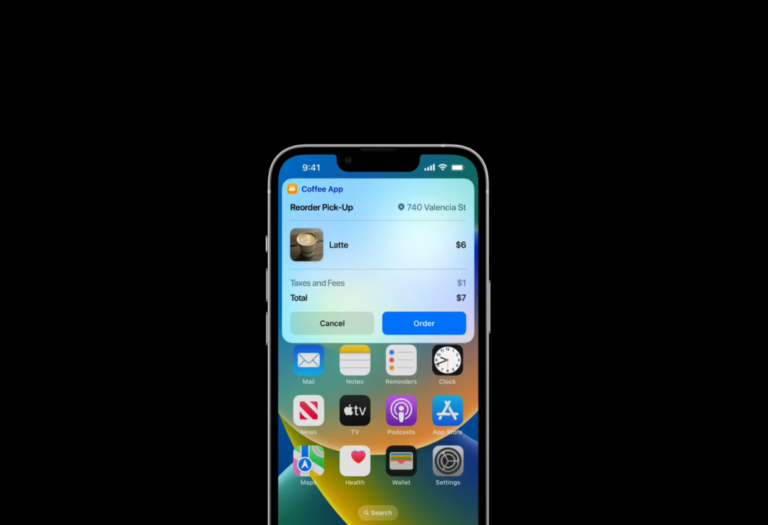